可以直接借助元素在存储器中的相对位置来表示数据元素之间的逻辑关系: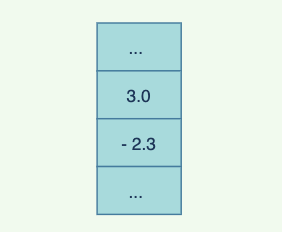而链式结构,则是以**指针**表示数据元素之间的逻辑关... 以及各种符号转换的开销,计算也更加高效。我们可以看到,下面负数参加运算的结果也是符合补码的规则的:```txt 00100011 35 + 11011101 -35------------------------- 00000000 ...
版本diff,以及潜在问题扫描检查有着极其重大的意义!上述是 SonarQube 做 CodeReview 的效果,此伪代码存在 BUG 大名鼎鼎的空指针!!! 而换成 AI 工具,我们来看一下ChatGPT如何随机应...
并不需要再检查这个对象的具体类型。sonic-JIT 的核心思想就是:**将模型解释与数据处理逻辑分离,让前者在“编译期”固定下来**。这种思想也存在于标准库和某些第三方 JSON 库,如 json-iterator 的函数组装模式... 不但可以让转换过程更加简单,甚至可以实现按需加载(lazy-load)——这便是 sonic-ast 的核心逻辑:**它是一种 JSON 在 Go 中的编解码对象,用** **node** **{type, length, pointer} 表示任意一个 JSON 数据节点,并结...
转换为机器特定指令集,这使得 eBPF 程序的运行效率与本机内核代码几乎一样高效,并且整个插桩过程对应用程序来说都是无感知、无侵入的。优秀的性能和无侵入的接入方式,很好地回答了前文提到的**接入成本**的问题。... ## **eBPF 具备全栈深度观测潜力**除了提供了很多预定义的 Hook 之外,eBPF 还允许我们创建内核探针 (kprobe) 或用户探针 (uprobe) 来将 eBPF 程序附加到内核或用户应用程序中的几乎任何位置。如下图所示,工程师...
奇葩说中的老师演讲大部分也在两个小时时间短的我可能花费了五个小时,时间长的我整整花费了三天时间去理解转换记录到文档中.....这些老师的课程虽然时间很短暂只有两个小时左右,但是对于一个小白的我来说,是打开... 而是希望自己以后碰到问题碰到场景的时候可以快速定位到文档,找寻一些其他的解决方案,并且更新自己不同时间段的不同理解### 迷茫阶段从上面的图中可以看到11月中下旬的时候已经没有更新了。经历了三个多月的疯狂...
下文中部分概念也会来自该视频资料。## App 启动类型App 启动过程有三种:冷启动、温启动 / 暖启动、 恢复。Cold | Warm | Resume---------|----------|---------After reboot | Recently terminated | A... `Rebase` 就是在程序启动过程中根据 ASLR 随机地址值修改应用内存地址的过程。主要过程就是从 `__LINKEDIT`取出函数指针,根据偏移量修改函数指针,存入`__DATA` 中,Rebase 解决了**内部的符号引用**问题。`Bindin...
> 本文整理自字节跳动基础架构资深研发工程师王万兴在火山引擎开发者社区 Meetup 中的分享。大模型离线推理,是指在具有数十亿或数千亿参数的大规模模型上进行分布式推理的过程。相较于常规模型推理,在模型切分、数... 从上图中可以看到,过去几年机器学习领域的模型参数增长非常迅猛,而相比于模型参数的增长,GPU 算力的提升相对较慢,两者之间就形成了越来越大的 Gap。这就带来一个问题,在进行推理或者训练时,GPU 内存可能放不下,需要...
AudioProfileType csharp public enum bytertc.AudioProfileType音质档位 Defined in : IRTCVideo.cs 枚举值类型 值 说明 kAudioProfileTypeDefault 0 默认音质。服务器下发或客户端已设置的 RoomProfileType 的音质配置。 kAudioProfileTypeFluent 1 流畅音质。单声道,采样率为 16kHz,编码码率为 24kbps。流畅优先、低延迟、低功耗、低流量消耗,适用于大部分游戏场景,如 MMORPG、MOBA、FPS 等游戏中的小队语音、组队语音、国战...
从上图中可以看到,过去几年机器学习领域的模型参数增长非常迅猛,而相比于模型参数的增长,GPU 算力的提升相对较慢,两者之间就形成了越来越大的 Gap。这就带来一个问题,在进行推理或者训练时,GPU 内存可能放不下,需要... 所以我们通过定义一个 Task(Python 函数),使用 Object 进行分布式的数据传输。右侧是使用 Ray 上层的 Library 编程,通过 RayTrain 训练一个简单的机器学习模型。使用时需要先定义一个模型,这个过程和直接用 P...
关键问题分析几个方面作深入介绍,并将介绍图计算相关实践。 自研图数据库(ByteGraph)介绍 从数据模型角度看,图数据库内部数据是有向属性图,其 **基本元素是 Graph 中的点(Vert... 我们就从代码层面介绍下点边的数据类型。* 点(Vertex)**点是图数据库的基本元素,通常反映的是静态信息**。在 ByteGraph 中,点包含以下字段:,使用 Object 进行分布式的数据传输。右侧是使用 Ray 上层的 Library 编程,通过 RayTrain 训练一个简单的机器学习模型。使用时需要先定义一个模型,这个过程和直接用 Pyt...
C接口 函数列表1. 创建动作识别的句柄函数定义 c BEF_SDK_API bef_effect_result_tbef_effect_ai_action_recognition_create( const char * model_path bef_effect_handle_t * handle );参数说明 参数名 参数类型 ... 参数说明 参数名 参数类型 参数说明 handle bef_effect_handle_t Created action_recognition handle 已创建的骨骼句柄 image const unsigned char * Image base address 输入图片的数据指针 pixel_format bef_ai_...
链路缺乏整体优化的问题,使得 GPU 能够充分发挥其强大的并行计算能力,应用于各类视频 AI 场景。目前BMF主要应用于视频转码、视频抽帧、视频增强、视频分析、视频插帧、视频编辑、视频会议等众多领域,为用户提供高... 使用BMF可以很简单地实现视频格式之间的转换。我们可以添加编码、解码和过滤模块,构建一个从MP4到MKV的转码Pipeline。了解配置接口后,即可按需设置参数,如改变视频大小或帧率等。2. 视频编辑通过添加视频拼接...